티스토리 뷰
[1]리스트박스
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace _20listbox
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("일본 , 오사카");
listBox2.Items.Add("호주 , 아델레이드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
List<string> happiness = new List<string>
{
"핀란드","덴마크","아이슬란드","스위스","네덜란드","룩셈부르크","스웨덴","노르웨이","이스라엘","뉴질랜드"
};
listBox3.DataSource = happiness;
}
//이벤트 처리 메서드
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
ListBox lst = sender as ListBox;
txtSIndex1.Text = lst.SelectedIndex.ToString();
txtSItem1.Text = lst.SelectedItem.ToString();
}
private void listBox2_SelectedIndexChanged(object sender, EventArgs e)
{
ListBox l = sender as ListBox;
txtSIndex2.Text = l.SelectedIndex.ToString();
txtSItem2.Text = l.SelectedItem.ToString();
}
private void listBox3_SelectedIndexChanged(object sender, EventArgs e)
{
ListBox l = sender as ListBox;
txtSIndex3.Text = l.SelectedIndex.ToString();
txtSItem3.Text = l.SelectedItem.ToString();
}
}
}
리스트박로 GDP와 살기좋은 나라 순위 그리고 행복한 나라 순위를 출력하는 앱을 만들어보았다.
리스트박스를 총 세개를 만들어 주었는데
리스트박스1에는 GDP를 설정해주었다.
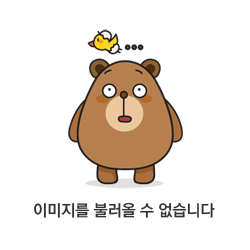
리스트박스1의 Items는 속성창을 사용하여 직접 collection에 값을 넣어주었다.
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("일본 , 오사카");
listBox2.Items.Add("호주 , 아델레이드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
listBox2.Items.Add("뉴질랜드 , 오클랜드");
리스트박스2에는 Items.Add()함수를 사용하여 일일히 값을 넣어주었고
List<string> happiness = new List<string>
{
"핀란드","덴마크","아이슬란드","스위스","네덜란드","룩셈부르크","스웨덴","노르웨이","이스라엘","뉴질랜드"
};
listBox3.DataSource = happiness;
리스트박스3에는 List클래스의 happiness를 string타입으로 선언하여
리스트 형식으로 값을 입력해주었고 Datasource에 happiness를 대입하여 주었다.
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
ListBox lst = sender as ListBox;
txtSIndex1.Text = lst.SelectedIndex.ToString();
txtSItem1.Text = lst.SelectedItem.ToString();
}
private void listBox2_SelectedIndexChanged(object sender, EventArgs e)
{
ListBox l = sender as ListBox;
txtSIndex2.Text = l.SelectedIndex.ToString();
txtSItem2.Text = l.SelectedItem.ToString();
}
private void listBox3_SelectedIndexChanged(object sender, EventArgs e)
{
ListBox l = sender as ListBox;
txtSIndex3.Text = l.SelectedIndex.ToString();
txtSItem3.Text = l.SelectedItem.ToString();
}
이 부분은 TB의 이벤트 선언부인데
listbox로부터 object클래스의 parameter인 sender형식의 값을 lst로 받아 IndexTB의 값에 선택된 값에 대한 인덱스와
아이템을 string형식으로 받아주었다.(sender에 대한 이해가 부족하여 더 공부해야할 것 같다.)
이를 따로 세번 함수를 작성하여준 뒤 실행하면
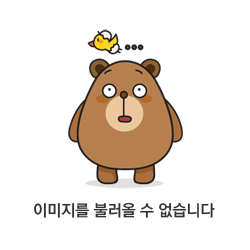
SelectedIndex에 선택된 값의 인덱스가
SelectedItem에 선택된 값이 출력된다.
[2]패널과 스크롤바
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace _20scroll
{
public partial class Form1 : Form
{
public Form1() //생성자 메서드
{
InitializeComponent();
this.Text = "ScrollBar Test";
this.BackColor = Color.LightSteelBlue;
panel1.BackColor = Color.Black;
txtR.Text = "0";
txtR.Text = "0";
txtR.Text = "0";
scrR.Maximum = 255 + 9;
scrG.Maximum = 255 + 9;
scrB.Maximum = 255 + 9;
}
private void scr_Scroll(object sender, ScrollEventArgs e)
{
txtR.Text = scrR.Value.ToString();
txtG.Text = scrG.Value.ToString();
txtB.Text = scrB.Value.ToString();
panel1.BackColor = Color.FromArgb(scrR.Value, scrG.Value, scrB.Value);
}
private void text_TextChanged(object sender, EventArgs e)
{
if(txtR.Text !="" && txtG.Text !="" && txtB.Text != "")
{
scrR.Value = int.Parse(txtR.Text);
scrG.Value = int.Parse(txtG.Text);
scrB.Value = int.Parse(txtB.Text);
}
}
}
}
패널과 리스트박스를 사용하여 스크롤바를 움직여 패널의 rgb값을 조정하여 색을 출력하는 코드를 작성해보았다.
public Form1() //생성자 메서드
{
InitializeComponent();
this.Text = "ScrollBar Test";
this.BackColor = Color.LightSteelBlue;
panel1.BackColor = Color.Black;
txtR.Text = "0";
txtR.Text = "0";
txtR.Text = "0";
scrR.Maximum = 255 + 9;
scrG.Maximum = 255 + 9;
scrB.Maximum = 255 + 9;
}
this포인터를 사용하여 현재 form의text(Title값)와 배경색을 설정해주었다.
panel의 배경색도 초기값은 black으로 설정해주었다.
txt들도 전부 0으로 설정해었고
스크롤바의 최대값을 255+9로 설정해주었다.(스크롤 바의 너비때문에 9를 더해준 것)
private void scr_Scroll(object sender, ScrollEventArgs e)
{
txtR.Text = scrR.Value.ToString();
txtG.Text = scrG.Value.ToString();
txtB.Text = scrB.Value.ToString();
panel1.BackColor = Color.FromArgb(scrR.Value, scrG.Value, scrB.Value);
}
txt들의 텍스트는 스크롤바의값에서 string형식으로 받아주었고
panel1의 배경색은 스크롤바들의 값을 int형식으로 받아 구성해주었다.
private void text_TextChanged(object sender, EventArgs e)
{
if(txtR.Text !="" && txtG.Text !="" && txtB.Text != "")
{
scrR.Value = int.Parse(txtR.Text);
scrG.Value = int.Parse(txtG.Text);
scrB.Value = int.Parse(txtB.Text);
}
}
위 코드로 텍스트들이 빈칸이 아닐 때 텍스트의 값을 받아 스크롤바의 값을 설정해준다
위 코드는 TB에 값을 입력하여 색을 바꾸는 것을 가능케하는 함수이다.
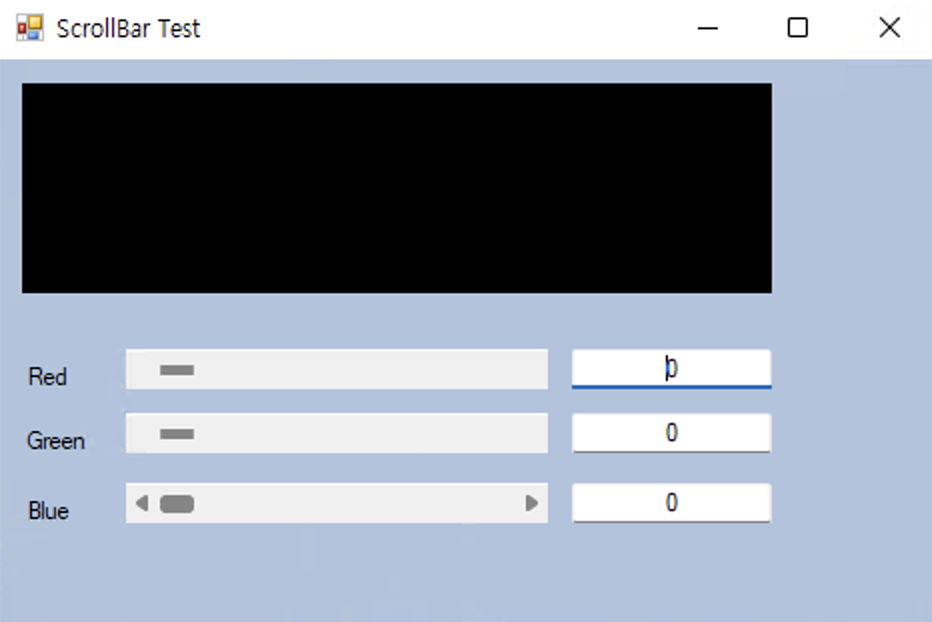
로드되었을 당시 폼의 화면이다.
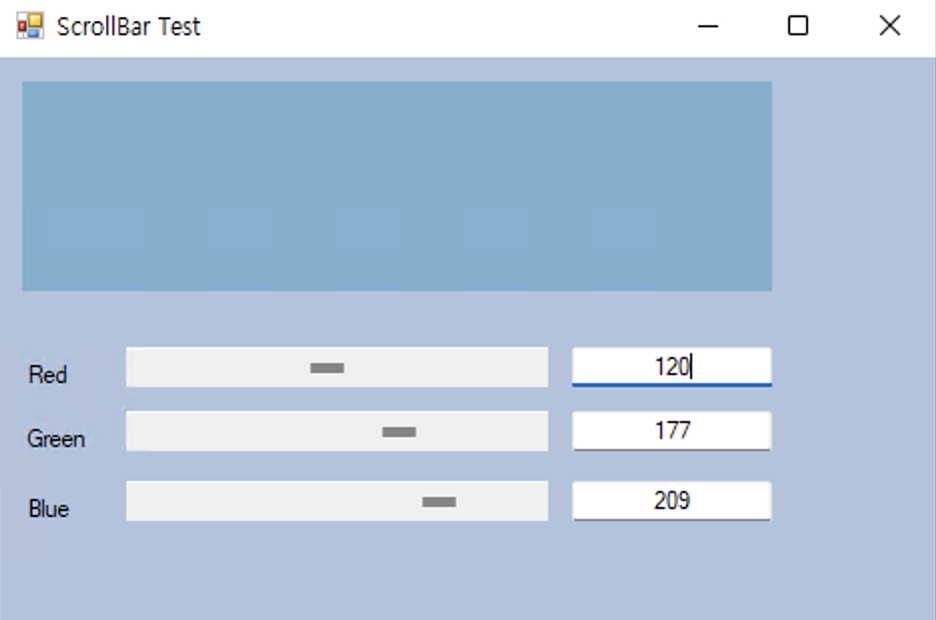
색을 바꿔주면 이렇게 변한다.
'VS-02분반수업' 카테고리의 다른 글
VS02(22-04-06) (0) | 2022.04.09 |
---|---|
VS02(22-04-01) (0) | 2022.04.08 |
VSP02(2022-03-23) (0) | 2022.03.31 |
VSP02(2022-03-16) (0) | 2022.03.23 |
VSP02(2022-03-18) (0) | 2022.03.22 |