티스토리 뷰
[1]전화번호부
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.OleDb;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace _32_PhoneBook2
{
public partial class Form1 : Form
{
OleDbConnection conn = null;
OleDbCommand comm = null;
OleDbDataReader reader = null;
string connStr = @"Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\Users\choi\Documents\Studb.accdb";
public Form1()
{
InitializeComponent();
Showstudent();
}
void Showstudent()
{
if (conn == null)
{
conn = new OleDbConnection(connStr);
conn.Open();
}
string sql = "SELECT * FROM Studb";
comm = new OleDbCommand(sql, conn);
reader = comm.ExecuteReader();
while (reader.Read())
{
string x = "";
x += reader["ID"] + "\t";
x += reader["sid"] + "\t";
x += reader["sname"] + "\t";
x += reader["phone"];
lbStudent.Items.Add(x);
}
reader.Close();
conn.Close();
conn = null;
}
private void button2_Click(object sender, EventArgs e)
{
if (textBox2.Text == "" || textBox3.Text == "" || textBox4.Text == "")
return;
ConnOpen();
string sql = string.Format("INSERT INTO studb(sid, sname, phone) VALUES({0}, '{1}', '{2}')", textBox2.Text, textBox3.Text, textBox4.Text);
//학번이 8자리 이상이어야 \t가 작동함
comm = new OleDbCommand(sql, conn);
int x = comm.ExecuteNonQuery();
if (x == 1)
MessageBox.Show("정상삽입");
ConnClose();
}
private void ConnClose()
{
conn.Close();
conn = null;
lbStudent.Items.Clear();
Showstudent();
}
private void ConnOpen()
{
if (conn == null)
{
conn = new OleDbConnection(connStr);
conn.Open();
}
}
private void lbStudent_SelectedIndexChanged_1(object sender, EventArgs e)
{
ListBox lb = (ListBox)sender;
if (lb.SelectedItem == null)
return;
string[] s = lb.SelectedItem.ToString().Split('\t');
//MessageBox.Show(lb.SelectedItem.ToString());
textBox1.Text = s[0];
textBox2.Text = s[1];
textBox3.Text = s[2];
textBox4.Text = s[3];
}
private void button5_Click(object sender, EventArgs e)
{
if (textBox1.Text == "")
return;
ConnOpen();
string sql = string.Format("DELETE FROM studb WHERE ID={0}" , textBox1.Text);
comm= new OleDbCommand(sql, conn);
int x = comm.ExecuteNonQuery(); //논쿼리의 리턴값은 int임 true면 1반환
if (x == 1)
MessageBox.Show("정상적으로 삭제되었습니다.");
ConnClose();
}
private void button6_Click(object sender, EventArgs e)
{
ConnOpen();
string sql = string.Format("UPDATE studb SET sid={0},"
+ "sname='{1}',phone='{2}'" + "WHERE ID={3}", textBox2.Text, textBox3.Text, textBox4.Text, textBox1.Text);
comm=new OleDbCommand(sql, conn);
int x = comm.ExecuteNonQuery();
if (x == 1)
MessageBox.Show("수정 성공!");
ConnClose();
}
private void button3_Click(object sender, EventArgs e)
{
if (textBox2.Text == "" && textBox3.Text == "" && textBox4.Text == "")
return;
ConnOpen();
string sql = "SELECT * FROM studb ";
if (textBox2.Text != "")
sql += "WHERE sid = " + textBox2.Text;
else if(textBox3.Text !="")
sql += "WHERE sname = '" + textBox3.Text + "'";
else if (textBox4.Text != "")
sql += "WHERE phone = '" + textBox4.Text + "'";
MessageBox.Show(sql);
comm = new OleDbCommand(sql, conn);
lbStudent.Items.Clear();
reader = comm.ExecuteReader();
while (reader.Read())
{
string x = "";
x += reader["ID"] + "\t";
x += reader["sid"] + "\t";
x += reader["sname"] + "\t";
x += reader["phone"];
lbStudent.Items.Add(x);
}
reader.Close();
conn.Close();
conn = null;
}
private void button4_Click(object sender, EventArgs e)
{
textBox1.Text = "";
textBox2.Text = "";
textBox3.Text = "";
textBox4.Text = "";
}
private void button1_Click(object sender, EventArgs e)
{
lbStudent.Items.Clear();
Showstudent();
}
private void button7_Click(object sender, EventArgs e)
{
Close();
}
}
}
button5(삭제버튼)부터 구현을 시작하겠습니다.
private void button5_Click(object sender, EventArgs e)
{
if (textBox1.Text == "")
return;
ConnOpen();
string sql = string.Format("DELETE FROM studb WHERE ID={0}" , textBox1.Text);
comm= new OleDbCommand(sql, conn);
int x = comm.ExecuteNonQuery(); //논쿼리의 리턴값은 int임 true면 1반환
if (x == 1)
MessageBox.Show("정상적으로 삭제되었습니다.");
ConnClose();
}
ID.Textbox가 비어있으면 빈 값을 리턴한다.
ConnOpen()으로 연결을 진행해준 뒤
string타입 sql에 삭제하는 문법의 문자열을 포맷하여 저장해준다.
comm을 sql과 conn을 기반으로 설정해준 뒤
x에 int형을 반환하는 ExecutenonQuery()의 값을 저장해준다.
x==1이면 메시지박스를 띄워줌
ConnClose()
private void button6_Click(object sender, EventArgs e)
{
ConnOpen();
string sql = string.Format("UPDATE studb SET sid={0},"
+ "sname='{1}',phone='{2}'" + "WHERE ID={3}", textBox2.Text, textBox3.Text, textBox4.Text, textBox1.Text);
comm=new OleDbCommand(sql, conn);
int x = comm.ExecuteNonQuery();
if (x == 1)
MessageBox.Show("수정 성공!");
ConnClose();
}
ConnOpen()으로 연결해준 뒤
sql에 위와같이 SQL문법의 문장을 문자열포매팅하여 저장해준다.
그리고 위와같이 연결해준다.
private void button3_Click(object sender, EventArgs e)
{
if (textBox2.Text == "" && textBox3.Text == "" && textBox4.Text == "")
return;
ConnOpen();
string sql = "SELECT * FROM studb ";
if (textBox2.Text != "")
sql += "WHERE sid = " + textBox2.Text;
else if(textBox3.Text !="")
sql += "WHERE sname = '" + textBox3.Text + "'";
else if (textBox4.Text != "")
sql += "WHERE phone = '" + textBox4.Text + "'";
MessageBox.Show(sql);
comm = new OleDbCommand(sql, conn);
lbStudent.Items.Clear();
reader = comm.ExecuteReader();
while (reader.Read())
{
string x = "";
x += reader["ID"] + "\t";
x += reader["sid"] + "\t";
x += reader["sname"] + "\t";
x += reader["phone"];
lbStudent.Items.Add(x);
}
reader.Close();
conn.Close();
conn = null;
}
sid.Text , sname.Text , phone.Text 모두가 빈칸일 시 리턴함
string sql = "SELECT * FROM studb ";
if (textBox2.Text != "")
sql += "WHERE sid = " + textBox2.Text;
else if(textBox3.Text !="")
sql += "WHERE sname = '" + textBox3.Text + "'";
else if (textBox4.Text != "")
sql += "WHERE phone = '" + textBox4.Text + "'";
sql을 "SELECT * FROM studb "로 지정해준 뒤
textbox2~4까지 비교해주면서
빈칸이 아닌 값이 있을 시 sql += "WHERE sid = " + textBoxN.Text 로 N번째 값에대한 정보를 추가로
where문을 사용하여 저장해준다.
MessageBox.Show(sql);
계속 오류가 나서 값이 잘 들어갔는지 확인하기 위해 messagebox를 사용하여 확인해본다.
comm = new OleDbCommand(sql, conn);
lbStudent.Items.Clear();
reader = comm.ExecuteReader();
다시 comm을 설정해준 뒤 리스트박스의 아이템을 clear()로 모두 지워준 뒤 reader를 comm값을 토대로 한
ExexuteReader로 지정해준다.
while (reader.Read())
{
string x = "";
x += reader["ID"] + "\t";
x += reader["sid"] + "\t";
x += reader["sname"] + "\t";
x += reader["phone"];
lbStudent.Items.Add(x);
}
reader.Close();
conn.Close();
conn = null;
reader.Read()가 true일 때 = 읽을 값이 존재할 때
string x 를 ""로 지정해준 뒤
\t로 구분한 ID , sid , sname , phone값을
리스트박스에 Add해준다.
private void button4_Click(object sender, EventArgs e)
{
textBox1.Text = "";
textBox2.Text = "";
textBox3.Text = "";
textBox4.Text = "";
}
private void button1_Click(object sender, EventArgs e)
{
lbStudent.Items.Clear();
Showstudent();
}
private void button7_Click(object sender, EventArgs e)
{
Close();
}
button4 = clear버튼
button1 = ViewAll버튼
button7 = 종료버튼
clear버튼은 모든 텍스트박스들을 ""로 바꿔준다.
viewall버튼은 리스트박스의 내용을 showstudent()함수를 사용하여 리로드 해준다.
종료버튼은 Close()를 사용하여 프로그램을 종료해준다.
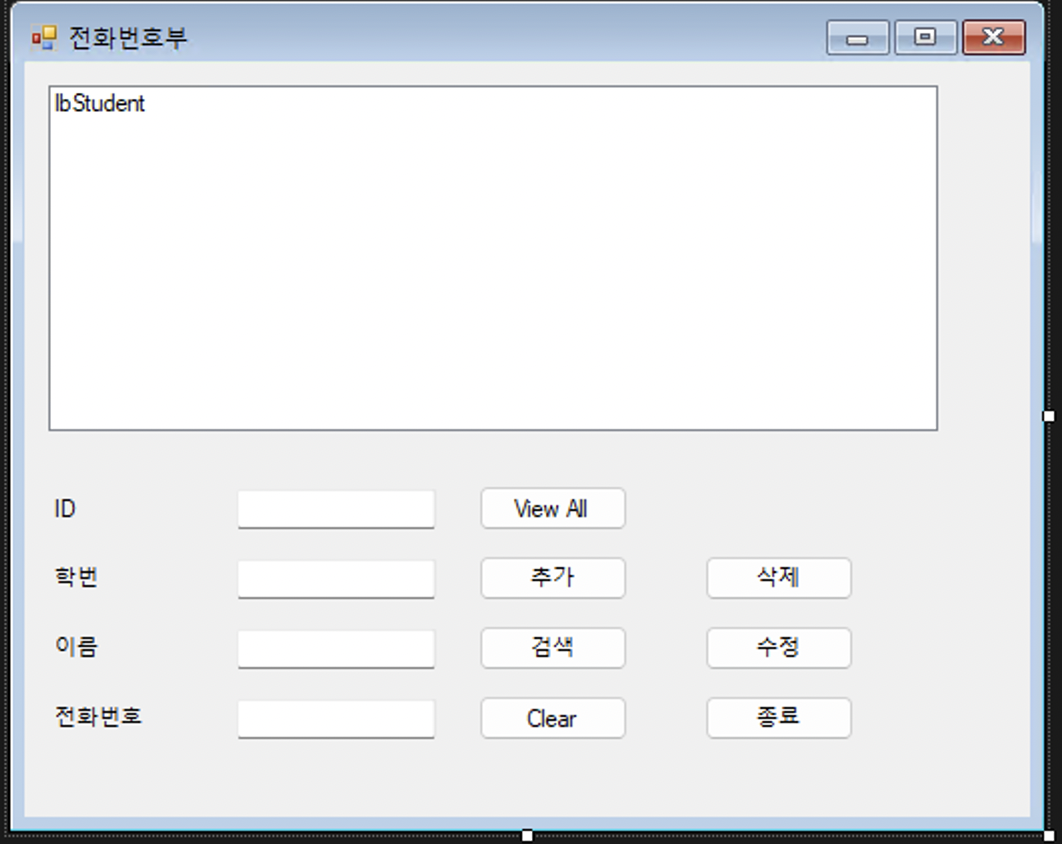
여기까지 전화번호부 프로그램을 마칩니다.
'VS-02분반수업' 카테고리의 다른 글
VP02(22-05-13) (0) | 2022.05.17 |
---|---|
VP02(22-05-11) (0) | 2022.05.16 |
VP(22-05-04) (0) | 2022.05.08 |
VS02(22-05-01) (0) | 2022.05.02 |
VS02(22-04-15) (0) | 2022.04.20 |